ASP.NET Core QR Code Barcode with a .NET Standard/.NET Core DLL
This Visual Studio project illustrates how to generate a QR Code barcode in ASP.NET Core with a .NET Standard/.NET Core DLL. The NETStandardQRCode.dll, available in nuget.org, validates the input data, generates barcode codewords and creates the necessary error correction characters. The following C# snippet illustrates how to use the DLL to generate a QR Code barcode.using Microsoft.AspNetCore.Mvc.RazorPages; using Net.ConnectCode.BarcodeFontsStandard2D; namespace ASPNETCoreQRCodeSample.Pages { public class IndexModel : PageModel { public string Message { get; set; } public void OnGet() { QR qrCode = new QR("12345678","M",8); string output=qrCode.Encode(); Message = output; } } }
Parameters
Input Data: elementBarcode.innerHTML (or any other input string)
Error Correction Level: "L" ("L", "M", "Q" or "H")
L - Allows recovery of up to 7% data loss
M - Allows recovery of up to 15% data loss
Q - Allows recovery of up to 25% data loss
H - Allows recovery of up to 30% data loss
Mask: 8 (0 to 7 or 8 for Auto)
The purpose of a mask pattern is to make the QR code easier for a QR scanner to read.
Text Output
The NETStandardQRCode.dll generates a text output for easy integration into your application as shown below:
111111100110001111111 100000101101001000001 101110100001101011101 101110100101101011101 101110101011101011101 100000100001001000001 111111101010101111111 000000000000000000000 101010100010100010010 010101001001010100001 101001100011011101000 001011001101110111001 101100101111011100011 000000001110001001010 111111100000100010001 100000100100001000011 101110101110101011001 101110100001010101010 101110101101011100101 100000100111110111000 111111101101011100101
A 1 represents a black square and a 0 represents a white square. Each row ends with an OS environment newline, "\r\n" for non-Unix platforms or "\n" for Unix platforms.
Rendering the QR Code Barcode
The output can be easily rendered on a HTML5 Canvas through a JavaScript function or displayed directly with a Web Open Font Format (WOFF) barcode font. For example, if you have the following HTML5 Canvas tag in your HTML web page.
<canvas id="barcodeCanvas" width=300 height=300>@Model.Message</canvas>
You can use the following function in qrcodecanvas.js to render the barcode onto the Canvas.
<script> drawQROnCanvas("barcodeCanvas"); </script>
You will get the following QR Code Barcode that meets the strictest requirements required by the auto-id industry.
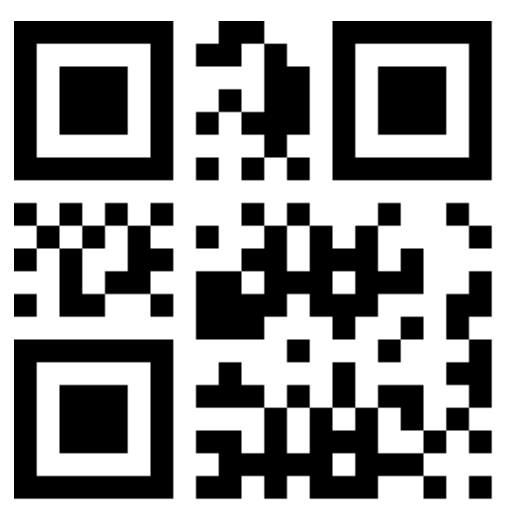
QR Code Barcode with .NET Standard DLL and Barcode Web Fonts
You can also use the Web Open Font Format (WOFF) QR Code fonts provided by ConnectCode to display the barcodes. For example, using the following div tag in your HTML web page:<div id="barcodeFonts" style="white-space: pre-line">@Model.Message</div>
You can use the Cascading Style Sheets (CSS) @font-face feature to specify an online font (the WOFF QR Code font) for display on web pages. Please refer to site.css for more details.
@font-face { font-family: CCodeQR; src: url("fonts/CCodeQR_Trial.eot"); src: local("CCodeQR_Trial"), url("fonts/CCodeQR_Trial.otf") format("opentype"), url("fonts/CCodeQR_Trial.woff") format("woff"); }
Next, apply the font-face to the barcodeFonts div element:
#barcodeFonts { font-weight: normal; font-style: normal; line-height: normal; font-family: 'CCodeQR', sans-serif; font-size: 12px; }
You will get the following QR Code Barcode generated using WOFF Barcode Font (W3C Compliant) that meets the strictest requirements required by the auto-id industry.
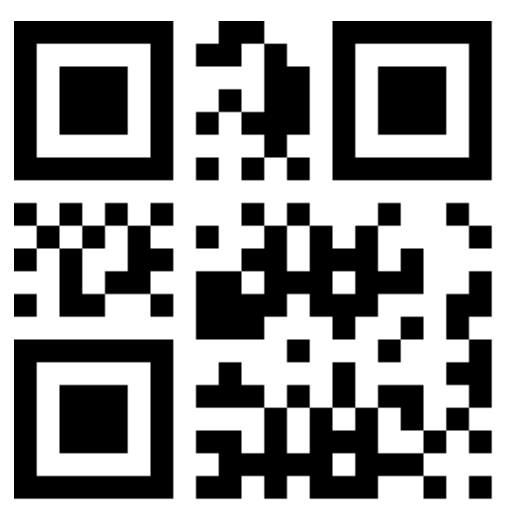
Supported Browsers and Platforms
ConnectCode Certified- Internet Explorer 11 (Windows 7/8/10)
- Microsoft Edge (Windows 8/10)
- Opera 40+ (Windows)
- Google Chrome 61+ (Windows)
- Google Chrome 61+ (OS X/macOS)
- Safari 5.1+ (OS X/macOS/Big Sur/Monterey)
- iOS Mobile Browser (iOS 10.0+)
- Android Mobile Browser (Android 4.0+)
- Mozilla Firefox (57+)
Please note that though our QR Code Barcode Web Fonts/HTML5 Canvas solution works on many more browsers, we currently only provide technical support for the above-mentioned certified browsers.
QR Code .NET Standard DLL
- .NET Standard 2.0+
Back to QR Code Barcode Fonts.