Create QR Code with a Component Object Model (COM) Library
This tutorial illustrates the use of a COM (Component Object Model) object library with a True Type Font (QR Code Barcode Font), provided in ConnectCode QR Code package, to create a ISO/IEC 18004:2015 standard-compliant QR Code in a .NET Windows Form application.Prerequisites
- ConnectCode QR Code package is installed
- QRCodeCOMLibrary.dll in the Resource\QRCodeCOMLibrary subdirectory of ConnectCode QR Code package.
The QR Code class library has been compiled with the "Register for COM interop" Visual Studio project property, exposing a COM-callable wrapper that enables COM interaction. - Visual Studio 2015/2017/2019
- Administrator Rights
Tutorial
1. Launch the Visual Studio Developer Command Prompt as Administrator from the Windows Start Menu.2. In the Developer Command Prompt, use the "cd" command to go to the QR Code COM Library folder.
cd C:\Program Files (x86)\ConnectCodeQRCode\Resource\QRCodeCOMLibrary (or ConnectCodeQRCodeTrial if you are using the Trial package)
3. Enter the following command in the Developer Command Prompt to use Regasm.exe to register the QRCode COMLibrary assembly for use with COM.
Regasm QRCodeCOMLibrary.dll /tlb:QRCodeCOMLibrary.tlb /codebase
Regasm.exe adds information about the class to the system registry so that COM clients can use the .NET Framework class transparently. The tlb option generates a type library defined within the assembly.
4. Launch Visual Studio. Create a new Windows Form project by clicking on "File->New Project", select a "Windows Forms App" and click on "Create" button.
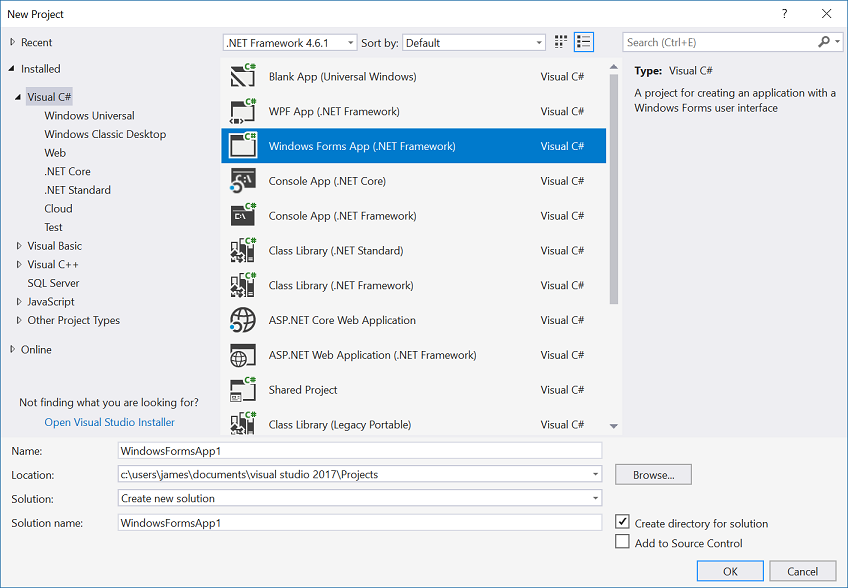
5. Double click on "Form1.cs" in the "Solution Explorer" and add a "Button" and a "RichTextBox" from the Visual Studio Toolbox. You should see your Windows Form similar to the screenshot below.
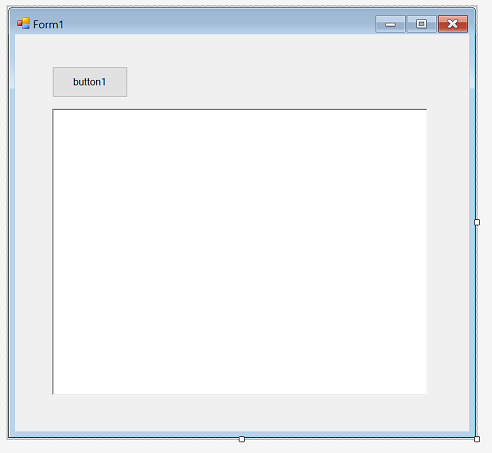
6. Right click on the "WindowsFormApp1" project in the "Solution Explorer" and select "Add->Existing Item". Navigate to the "C:\Program Files (x86)\ConnectCodeQRCode\" folder and select the "CCodeQR.ttf" font. If you are using the trial version, select the "CCodeQR_Trial.ttf" font instead.
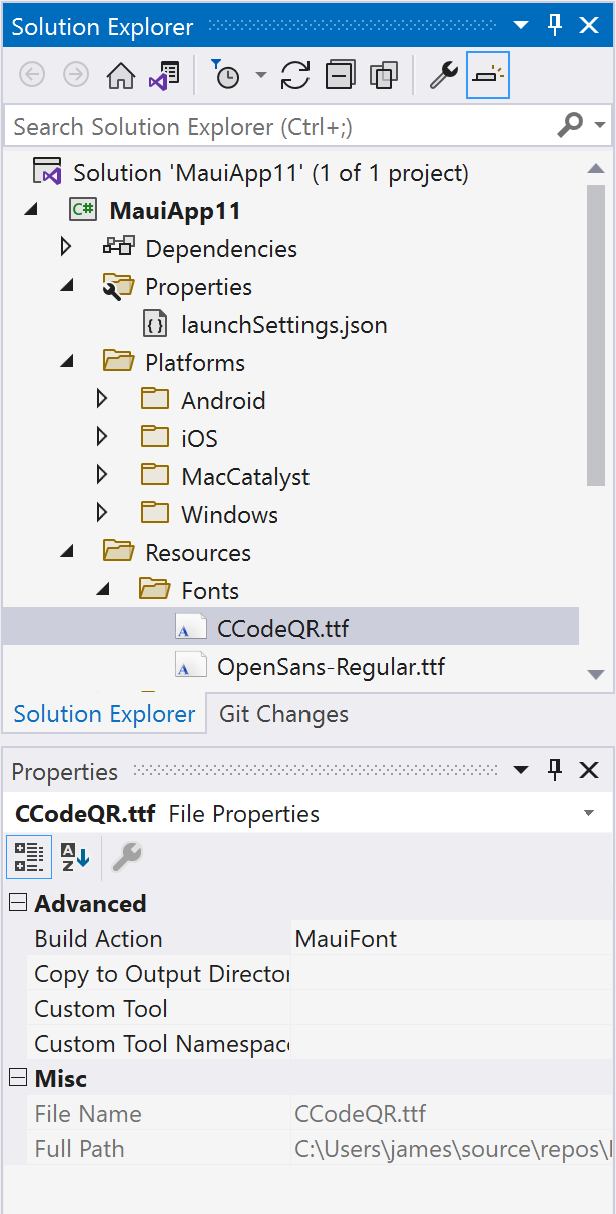
7. In "Solution Explorer", select the "CCodeQR.ttf" font and change the "Build Action" to "Content" and "Copy to Output Directory" to "Copy Always" in the Properties pane. This will ensure that Visual Studio deploy the QR Code barcode font for use with the Windows Form application.
8. Double click on "Form1.cs" in the "Solution Explorer". In the designer, double click on the Button. This will generate the button1_Click function in the editor. Enter the C# programming codes as shown below:
using System.Drawing.Text; . . . . private void button1_Click(object sender, EventArgs e) { try { Type comObjectType = Type.GetTypeFromProgID("Net.ConnectCode.QRCodeCOMLibrary"); dynamic theComObject = Activator.CreateInstance(comObjectType, false); //or //Guid myGuid = new Guid("5E206D5A-D9C2-45AA-BBFD-2E9B36AD437D"); //Type comObjectType = Type.GetTypeFromCLSID(myGuid); //dynamic theComObject = Activator.CreateInstance(comObjectType, false); string inputData = "12345678"; string ecl = "L"; //L, M, Q or H int mask = 8; //0 to 7 or 8 for Auto string result1 = theComObject.Encode_QRCode(inputData,ecl,mask); string result = result1; richTextBox1.Text = result; System.Diagnostics.Debug.WriteLine(result1); PrivateFontCollection pfc = new PrivateFontCollection(); pfc.AddFontFile("CCodeQR.ttf"); //pfc.AddFontFile("CCodeQR_Trial.ttf"); richTextBox1.Font = new Font(pfc.Families[0], 8, FontStyle.Regular); } catch (Exception ex) { System.Diagnostics.Debug.WriteLine(ex); }The C# function above creates a QR Code COM object and then uses it to generate a QR Code barcode with the input data "12345678". The result is placed in "richTextBox1" and the final output is displayed with the CCodeQR True Type font. When you run the application, click on the "Encode with COM" button, you should see the QR Code barcode as shown below .
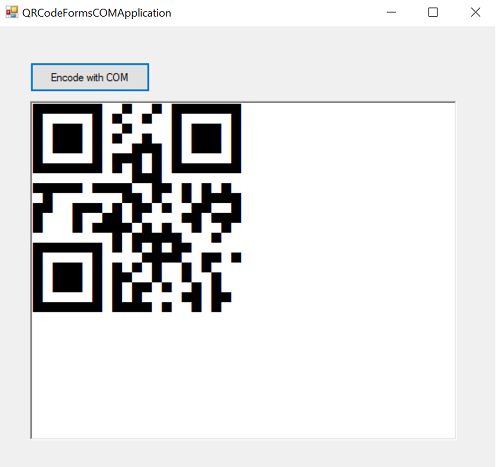
The "QRCodeCOMApplication" folder in "C:\Program Files (x86)\ConnectCodeQRCode\Resource" contains the full source code of the above application.