How to create barcodes in ASP.NET Core (Razor)?
ASP.NET Core
ASP.NET Core is an open-source web framework developed by Microsoft and the community that runs on different platforms such as Windows, Linux, and macOS. The framework uses the .NET Core runtime and enables you to easily build web apps, cloud services, IoT apps, and mobile backends.Tutorial on creating a Code 128 Barcode in ASP.NET Core
This tutorial illustrates how to create a Code 128 barcode in ASP.NET Core with barcode fonts and a .NET Core DLL available on nuget.org. A barcode generated using fonts is of the highest quality and meets the strictest requirements of the auto-id industry.Prerequisites
- .NET Core 3.0 SDK
- Visual Studio Code (or Visual Studio 2019 16.3 onwards)
- ConnectCode Barcode Software and Fonts package is installed. In this documentation, we assume you are using Windows for your web development. Please be assured that all fonts and .NET Core DLL can be used on other platforms such as macOS and Linux.
2. Launch Visual Studio Code, click on "File->Open Folder" and select the "ASPNETCoreBarcode" folder.
3. Click on "Terminal->New Terminal" and enter the following command in the "Terminal" console.
dotnet new webapp
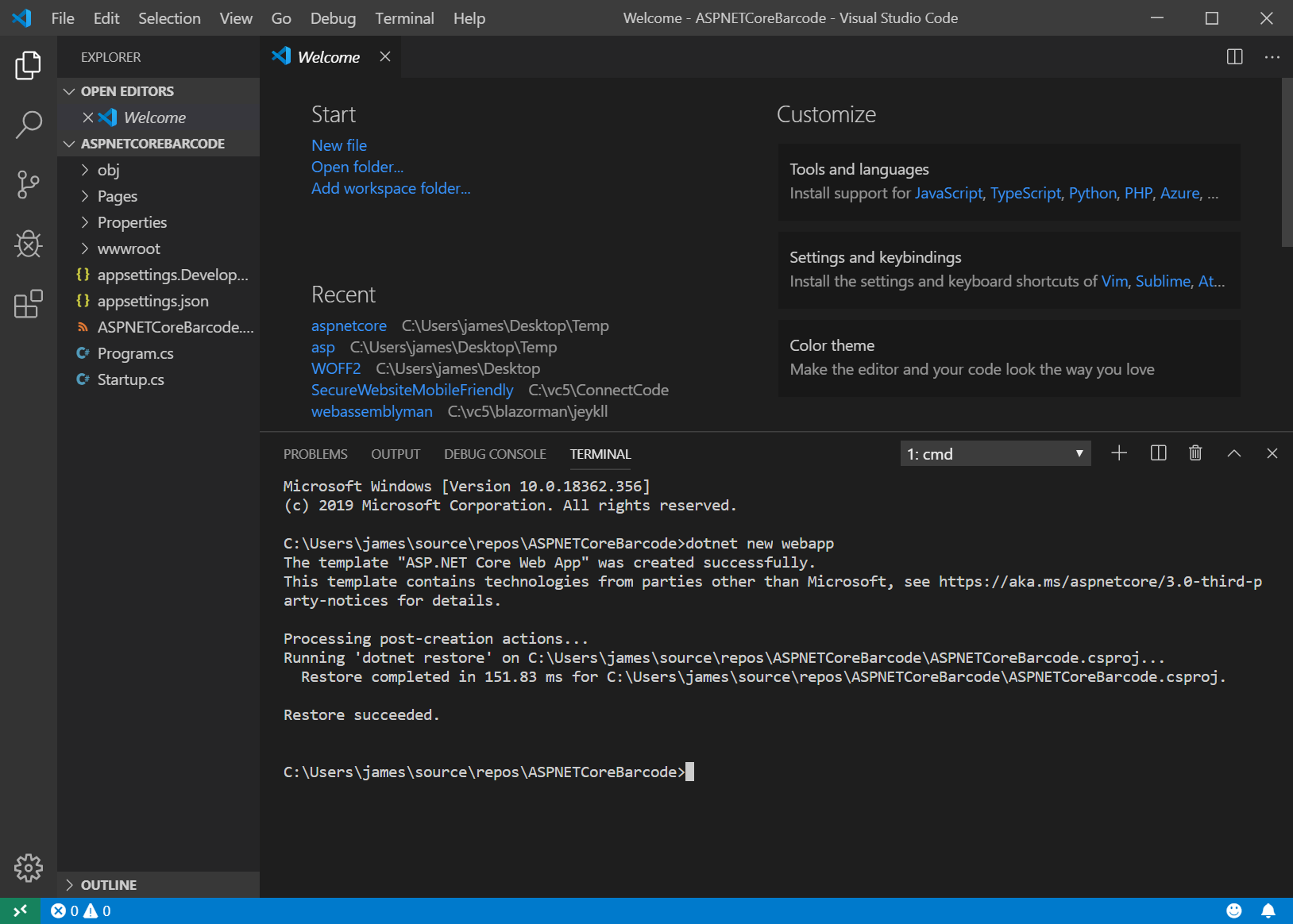
If the "dotnet" command is not available, please make sure you have installed .NET Core SDK. Next, restore the dependencies and tools of the project by entering the following command in the "Terminal" console.
dotnet restore
4. At this point, the ASP.NET Core web app project is ready to run. If you try to run and access it on HTTPS via "https://localhost:5001", you may see an error "Your connecttion to this site is not secure". This is because an untrusted SSL cert being used. To trust this SSL cert in Windows, simply enter the following command. This will add the certificate to the certificate store. For other operating systems, please check out the relevant documentation.
dotnet dev-certs https --trust
Click "Yes" when you are prompted to install the certificate. If you are using Visual Studio 2019 (or later), the above step of trusting the SSL certs are automatically performed.
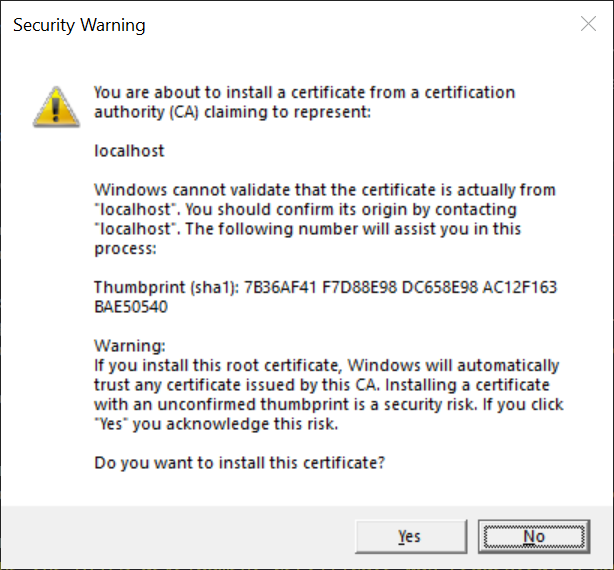
5. If you run the project using the command below.
dotnet run
You should see the following that indicates your project is created and run successfully. The generated web application contains a Home page ("Index.cshtml") and a Privacy page ("Privacy.cshtml"). The two pages use Razor, an ASP.NET Core programming syntax, for creating dynamic web pages.
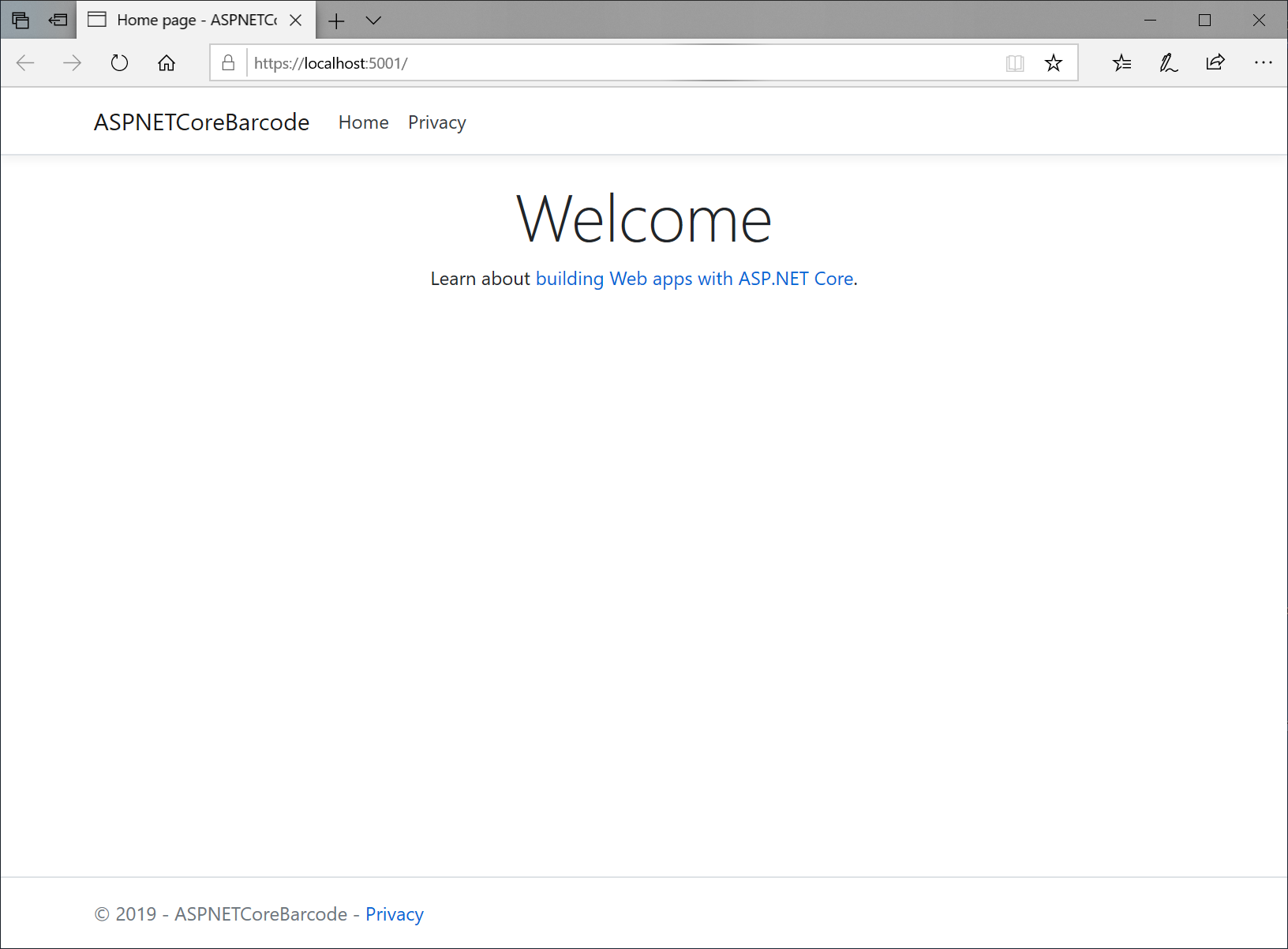
Enter "Ctrl-C" in the "Terminal" console to stop the web application.
6. In the "Terminal" console, enter the following command to add the "NETCoreBarcode.dll" from nuget.org.
dotnet add package NETCoreBarcode
The latest NETCoreBarcode.dll (v1.1) supports .NET Core 3.0. If you need a version that supports .NET Core 2.0, please use NETCoreBarcode.dll (v1.0). Make sure the package is added successfully as shown below.
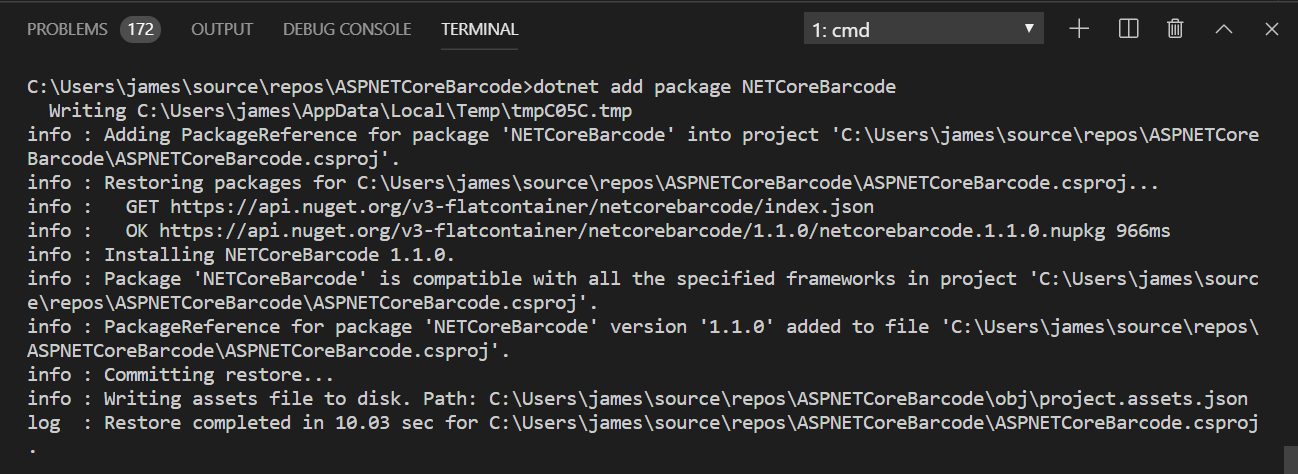
7. Next, we are going to edit the Home page of the web application to add our Code 128 barcode. Click on the "ASPNETCOREBARCODE->Pages->index.cshtml" file in Visual Studio Code Explorer and add a "div" element for "@Model.Barcode" as shown below. This element displays our barcode. The "BarcodeText" element is also needed to display the human readable text, commonly found at the bottom of a barcode.
<div class="text-center"> <h1 class="display-4">Welcome</h1> <p>Learn about <a href="https://docs.microsoft.com/aspnet/core"> building Web apps with ASP.NET Core</a>.</p> <div id="barcode">@Model.Barcode</div> <div id="barcode_text">@Model.BarcodeText</div> </div>
8. Click on the "index.cshtml.cs" class in Visual Studio Code Explorer. Add the following codes into the "index.cshtml.cs" class. Basically, the "OnGet" function uses the "BarcodeFonts" class in the "NETCoreBarcode.dll" to generate (also known as encode) a "Code128Auto" barcode. After encoding the input data, the output characters are stored in the "string Barcode" property. The output is a line of encoded text. When the text is applied with a Code 128 Barcode Font will give you a high-quality Code 128 barcode.
using Net.ConnectCode.Barcode; . . . [ViewData] public string Barcode {get; set;} [ViewData] public string BarcodeText {get; set;} public void OnGet() { BarcodeFonts bec= new BarcodeFonts(); bec.BarcodeType=BarcodeFonts.BarcodeEnum.Code128Auto; bec.Data="12345678"; bec.encode(); Barcode=bec.EncodedData; BarcodeText=bec.HumanText; }
9. Open "_Layout.cshtml" in Visual Studio Code and add the CSS definitions shown below. We are going to apply our barcode font to the "div" element defined in "index.cshtml". The "font-face" CSS tag specifies a Code 128 custom font and applies it to the "barcode" element. It is important to note that the font size of the "barcode" element is set to "32px". This is the default font size that we recommend you to start with. You can always increase or decrease the font size later to adjust the barcode to your desired size.
<head> . . . <STYLE TYPE="text/css" media="screen,print"> @@font-face { font-family: CCode128; src: url("/fonts/ConnectCode128_S3_Trial.ttf") format('TrueType') } #barcode { font-weight: normal; font-style: normal; line-height: normal; font-family: 'CCode128', sans-serif; text-align: center; font-size: 32px } #barcode_text { font-weight: normal; font-style: normal; line-height: normal; font-family: sans-serif; text-align:center; font-size: 24px } </STYLE> </head>
10. Finally, run the web application with the following command.
dotnet run
You should get the Code 128 barcode as shown below. To recap, we have generated a "string" using a .NET Core Barcode DLL and displayed it on our web page. The "string" when applied with our barcode font provides us with a high-quality barcode.
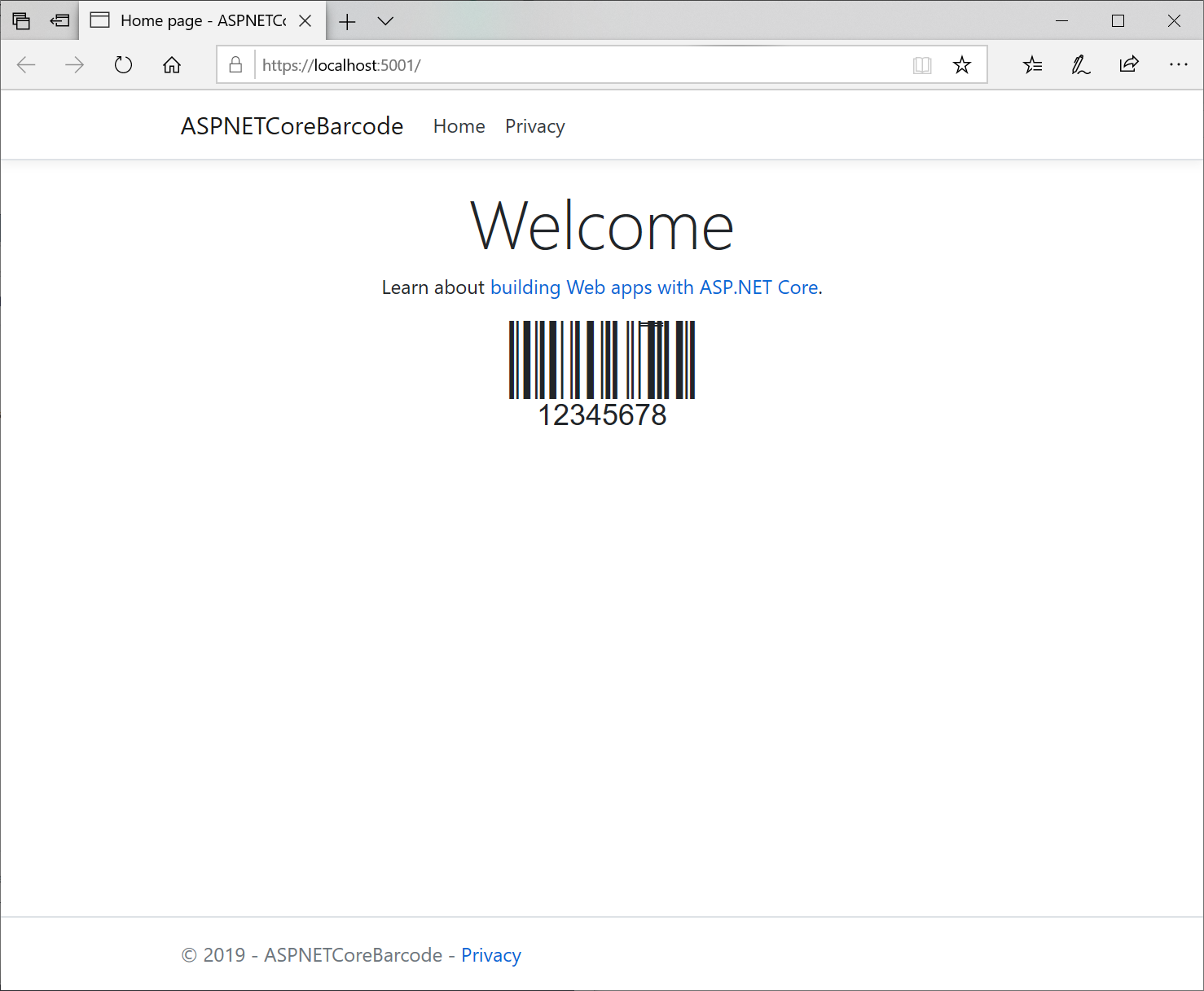